Another optimisation, locally a minor one, but also impacts ‘hasEvent’.
In the trial below, I always convert the name to lowercase. In most cases this will be needed because you can expect that the event that is looked for exists.
Then I check if the handler already exists in the table. If the handler is in the table, the event exists, doesn’t it?
By doing that I avoid a call to hasEvent, and only if the event does not exist yet, the call to hasEvent is made.
/**
* Returns the list of attached event handlers for an event.
* @param string $name the event name
* @return CList list of attached event handlers for the event
* @throws CException if the event is not defined
*/
public function _getEventHandlers($name)
{
if($this->hasEvent($name))
{
$name=strtolower($name);
if(!isset($this->_e[$name]))
$this->_e[$name]=new CList;
return $this->_e[$name];
}
else
throw new CException(Yii::t('yii','Event "{class}.{event}" is not defined.',
array('{class}'=>get_class($this), '{event}'=>$name)));
}
public function getEventHandlersAlt($name)
{
$name=strtolower($name);
if(isset($this->_e[$name])) {
return $this->_e[$name];
} elseif ($this->hasEvent($name)) {
return $this->_e[$name]=new CList;
}
else
throw new CException(Yii::t('yii','Event "{class}.{event}" is not defined.',
array('{class}'=>get_class($this), '{event}'=>$name)));
}
static $alternate=0;
public function getEventHandlers($name) {
self::$alternate=1-self::$alternate;
return self::$alternate?$this->getEventHandlersAlt2($name):$this->getEventHandlersAlt($name);
}
What’s the gain?
4201
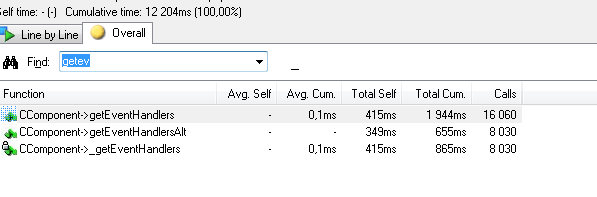
Well, there are only 8670 hasEvent calls versus 16060 calls - a gain of about 400ms.
The self of the function goes down from 412ms to 349ms. That is 15%, only 130ms.
So in all, a 530ms gain. The total cumulated for the getEventHandlers function has gone from 2.4 seconds on the first run to 1.2 seconds on the last run. That’s a nice 1.2 seconds gain on the 5.1 total or 24%. With a potential to gain 400ms more with the debug option.
That is confirmed by comparing the new profile with the original one.
4202
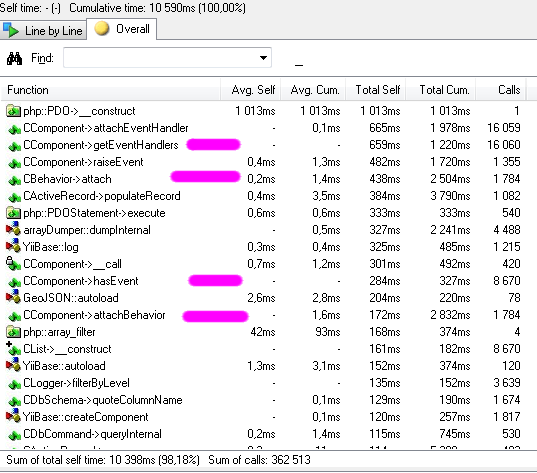
CComponent->attachBehavior has a total accumulated of 2.8 seconds in stead of 5.1 even if that is not entirely comparable because done in different runs.
php::PDO is at the head of the list too now which is preferable.
Next up is a look at attachEventHandler where 665ms are lost, and attachBehavior with a small loss of 168ms. [The arrayDumper seems to be worth a look as well as populateRecord].